4.10. The Java API
Last updated: 23 January 2013.
4.10.1. What is the Java API?
The Java API (Application Programming Interface) is composed of numerous utility classes and interfaces provided by the JDK, allowing developers to perform common tasks easily. The Java API covers a wide range of purposes and is divided into multiple packages like:
java.io for input and output management.
java.net for networking.
java.sql for database connectivity.
javax.swing for graphical user interfaces.
java.net for networking.
java.sql for database connectivity.
javax.swing for graphical user interfaces.
The Java API is provided with detailed documentation about the purpose and use of each package, class or interface. For example, for a given class, the documentation provides information about the fields, method signatures and details about the method parameters.
4.10.2. How to use the Java API documentation?
Go to the official website and download the API documentation. The download should be labeled Java SE x Documentation where x is the latest version. Unzip the downloaded file in the directory of your choice and open the following file with a web browser:
/directory_of_your_choice/docs/api/index.html for Linux and Mac users.
C:\directory_of_your_choice\docs\api\index.html for Windows users.
C:\directory_of_your_choice\docs\api\index.html for Windows users.
The page index.html should look something like this:

The page index.html is divided into 3 frames:
- The frame 1 lists all the packages of the Java API.
- The frame 2 lists by default all the classes of the entire Java API in alphabetical order. When you click a particular package in the frame 1, the frame 2 lists all the classes and interfaces of the selected package.
- The frame 3 lists by default all the packages of the Java API with a description of each package. When you select a class or an interface in the frame 2, the frame 3 shows the information of the selected class or interface. In particular, from top to bottom, you'll find the name of the package the selected class or interface belongs to, the class or interface hierarchy (list of super classes or super interfaces), summaries of its fields (if any), constructors (if any) and methods (if any) as well as details about the fields, constructors and methods.
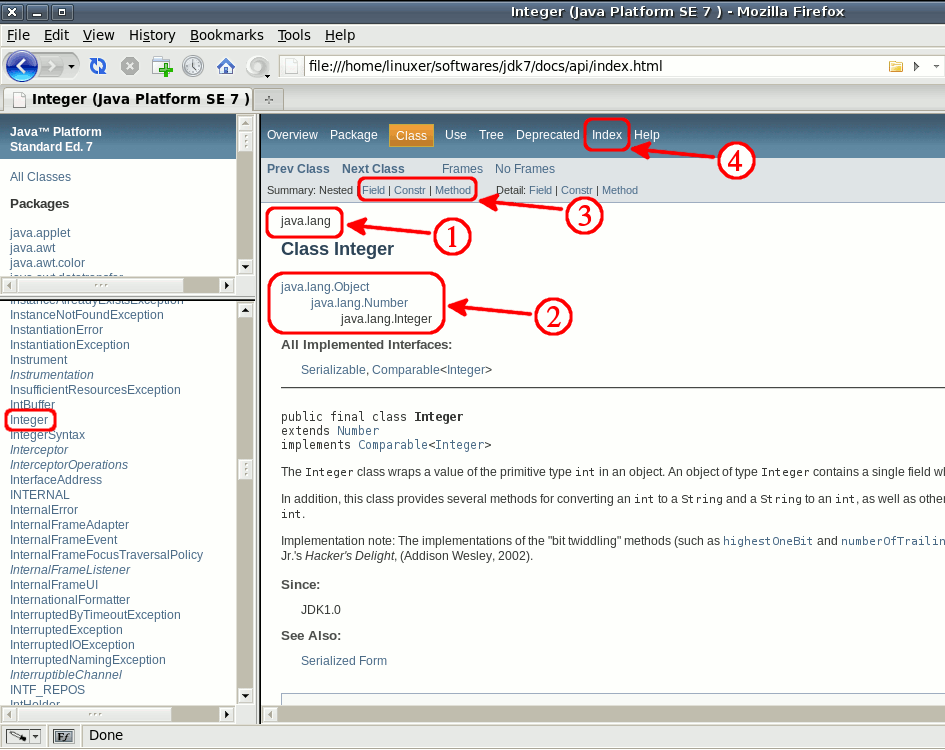
The class Integer that is given as an example is a wrapper for the primitive type int. For performance reasons, you should not use instances of the class Integer instead of the primitive type int unless you have a good reason to do so. Generally, the class Integer is used to convert String values to int values and vice versa.
The Java API provides other primitive wrappers such as Long, Float, Double, Character, Byte, Short and Boolean. Once again, you should not use instances of these classes instead of the corresponding primitive types for performance reasons (primitive wrappers require more memory and time). Nonetheless, the primitive wrappers are useful because of the utility methods they provide. For example, the class Character has a static method named isDigit(char ch) which returns a boolean indicating whether the passed character is a digit or not.
Now let's return to the example above and take a look at the main frame on the right hand side where some areas are outlined in red:
- The area 1 contains the package name. As you can see, the class Integer belongs to the package java.lang which is the only package that is automatically imported in all Java programs. As a result, if you want to use the class Integer, there is no need to import it. On the contrary, all the classes and interfaces belonging to the other packages of the API have to be imported explicitly.
- The area 2 shows the class hierarchy: the class Integer is a subclass of the class Number which is itself a subclass of the class Object which is the mother of all classes. Every class of the API and every class that is created by a developer is a subclass of the class Object.
When you start using the Java API, it helps to know that you can call the methods and access the fields of the super classes as well. In some situations, you might be expecting the current class to implement a certain functionality. If it is not the case (that is, you didn't find the appropriate method in the methods summaries), then you can look for that functionality in the super class. If you still didn't find it in the super class, then search in the class above the super class and so on. - The area 3 contains links to the fields, constructors and methods summaries.
- The area 4 is a link to the index which contains the list of all the methods, fields, constructors, classes and interfaces of the API in alphabetical order.
Another interesting class you could take a look at is the class String. In Java, a string literal like "Hello World" is an object (an instance of the class String) and the class String provides a number of very useful methods.
Likewise, the class System that you have seen in action in the previous tutorials is very commonly used, especially for printing to the standard output. Basically, the class System has a field named out which is the standard output stream. Since it is a static field, it can be accessed via System.out. Furthermore, the type of the field out is PrintStream and as you can see in the API, the class PrintStream has a method named println(String x) that I have used in the previous tutorials in statements like System.out.println("Hello World !");
As you can see, the API documentation does not tell you how its methods are implemented. Actually, the whole API is implemented in the JVM. All you need to know is how to call the methods of the API, leaving their implementation to the JVM.
The API presented here is the core Java API. There are third-party APIs that are not included in the JVM. Therefore, if you want to use third-party APIs, you need to download their implementation and documentation. Those additional APIs are used for various purposes like database connection pooling or XML processing as you will see in the next tutorials.
If you often search the API documentation like the vast majority of Java developers (even the experienced ones), you will probably come across deprecated methods such as Character.isJavaLetter. Deprecated methods are methods that developers should no longer use because they are not safe or they have been replaced by improved methods. If you use a deprecated method, the compiler will display a warning. Interfaces, classes, constructors, fields and methods can be deprecated.
4.10.3. How to make your own API documentation?
The Javadoc tool that is included in the JDK allows you to create your own API documentation from your source code. All you have to do is write javadoc-style comments before the fields, methods, classes and interfaces of your choice. A javadoc-style comment must be written as shown below:
/**
* This javadoc-style comment will appear in the generated API documentation.
* As you can see, the comment starts with /** (two asterisks instead of one).
*/
* This javadoc-style comment will appear in the generated API documentation.
* As you can see, the comment starts with /** (two asterisks instead of one).
*/
For example, let's add basic javadoc-style comments to the following class named MyClass containing a single method named myMethod that takes arguments and returns a boolean:
/**
* The description of the class MyClass goes here<br/>
* The description can span several lines<br/>
* You must use HTML tags to format your text<br/>
* @author yourName
*
*/
public final class MyClass {
/**
* @param argument1 (int) - the description of argument1 goes here
* @param argument2 (char) - the description of argument2 goes here
* @param argument3 (double) - the description of argument3 goes here
* @return (boolean) - the description of the returned value goes here
*/
public boolean myMethod(int argument1, char argument2, double argument3) {
// The code of myMethod goes here
}
}
* The description of the class MyClass goes here<br/>
* The description can span several lines<br/>
* You must use HTML tags to format your text<br/>
* @author yourName
*
*/
public final class MyClass {
/**
* @param argument1 (int) - the description of argument1 goes here
* @param argument2 (char) - the description of argument2 goes here
* @param argument3 (double) - the description of argument3 goes here
* @return (boolean) - the description of the returned value goes here
*/
public boolean myMethod(int argument1, char argument2, double argument3) {
// The code of myMethod goes here
}
}
To generate the documentation, open a command prompt, change to the directory where your Java source files reside and type the following command:
javadoc MyClass.java -d destination_directory
The option -d is to specify a destination directory for the generated HTML files. When the files are generated, open the file index.html with a web browser.
Note that you can also generate documentation for an entire package this way:
javadoc mypackage -d destination_directory
To indicate that a program element in your own code is deprecated, just add the @deprecated tag to the element's documentation. Here is an example:
/**
* @deprecated
* This method is deprecated because ...
*/
public void yourDeprecatedMethod(){
// ...
// ...
}
* @deprecated
* This method is deprecated because ...
*/
public void yourDeprecatedMethod(){
// ...
// ...
}
You are here :
JavaPerspective.com >
Beginner Tutorials >
4. Object-Oriented Concepts >
4.10. The Java API
Next tutorial : JavaPerspective.com > Beginner Tutorials > 4. Object-Oriented Concepts > 4.11. Exceptions
Next tutorial : JavaPerspective.com > Beginner Tutorials > 4. Object-Oriented Concepts > 4.11. Exceptions