JavaPerspective.com >
Intermediate Tutorials >
5. Graphical User Interfaces >
5.11. Formatted Text Fields
5.11. Formatted Text Fields
Last updated: 25 January 2013.
This tutorial will show you how to use formatted text fields in Java.
A formatted text field is an instance of the class JFormattedTextField which is a direct subclass of JTextField. Therefore, a formatted text field is like a normal text field except that is controls the validity of the characters the user types in. More precisely, a formatted text field is associated with a formatter that specifies the characters the user can enter. The JDK provides built-in formatters for dates and numbers. Additionally, a mask formatter is also provided by the JDK. With a mask formatter, you can specify a combination of valid characters. For example, the mask ##-##-## is to specify that a valid entry must be 3 groups of 2 digits separated by hyphens.
A formatter is a subclass of the class Format. Typically, to build a formatted text field, you create a formatter, customize it if necessary and call the constructor JFormattedTextField(Format format) which takes an argument of type Format. As an example, the following sample builds a formatted text field in which the user can only enter decimal numbers with at most 2 fraction digits:
// Create the formatter
NumberFormat twoFractionDigitsFormat = NumberFormat.getNumberInstance();
// Customize the formatter
twoFractionDigitsFormat.setMaximumFractionDigits(2);
// Build the formatted text field
JFormattedTextField textField = new JFormattedTextField(twoFractionDigitsFormat);
NumberFormat twoFractionDigitsFormat = NumberFormat.getNumberInstance();
// Customize the formatter
twoFractionDigitsFormat.setMaximumFractionDigits(2);
// Build the formatted text field
JFormattedTextField textField = new JFormattedTextField(twoFractionDigitsFormat);
A key point to note when using a formatted text field is that there is a difference between the displayed text returned by the method getText and the value returned by the method getValue. The text changes as you type whereas the value does not change unless one of the following methods is called: setValue(Object value) or commitEdit. The latter is called automatically in two occasions: when you hit enter while the formatted text field has the focus and when the formatted text field loses the focus.
A call to the method setText(String t) inherited from the class JTextComponent updates the displayed text but does not affect the value of the formatted text field. Therefore, you should use the methods getValue and setValue(Object value) to manipulate a formatted text field instead of the methods getText and setText(String t) inherited from the class JTextComponent. If you want to make sure that the value of a text field is identical to the displayed text, you can call the method commitEdit explicitly before calling the method getValue.
The picture shown below is a frame containing several formatted text fields:
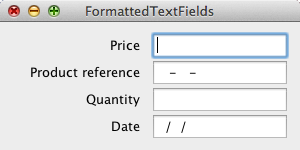
Each formatted text field is described by a label facing it:
- price must be a decimal number than can have at most 2 fraction digits.
- Product reference allows 3 groups of 3 digits separated by hyphens.
- Quantity must be an integer with a maximum of 2 digits.
- Date lets the user enter digits representing a date in the form dd/MM/yyyy (the day spans 2 digits, the month also spans 2 digits and the year spans 4 digits).
The code follows:
import java.awt.GridLayout;
import java.text.NumberFormat;
import java.text.ParseException;
import javax.swing.BorderFactory;
import javax.swing.JFormattedTextField;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
import javax.swing.text.MaskFormatter;
public final class FormattedTextFields extends JFrame {
public FormattedTextFields() throws ParseException {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() throws ParseException {
// Set up the content pane
JPanel contentPane = new JPanel(new GridLayout(0, 2));
contentPane.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
// Set up the price label
JLabel priceLabel = new JLabel("Price", SwingConstants.TRAILING);
priceLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(priceLabel);
// Set up the price formatted text field
NumberFormat priceFormat = NumberFormat.getNumberInstance();
priceFormat.setMaximumFractionDigits(2);
JFormattedTextField priceTextField = new JFormattedTextField(priceFormat);
priceTextField.setColumns(10);
contentPane.add(priceTextField);
// Set up the product reference label
JLabel productReferenceLabel = new JLabel("Product reference", SwingConstants.TRAILING);
productReferenceLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(productReferenceLabel);
// Set up the product reference formatted text field
MaskFormatter productReferenceFormat = new MaskFormatter("###-###-###");
JFormattedTextField productReferenceTextField = new JFormattedTextField(productReferenceFormat);
productReferenceTextField.setColumns(10);
contentPane.add(productReferenceTextField);
// Set up the quantity label
JLabel quantitylLabel = new JLabel("Quantity", SwingConstants.TRAILING);
quantitylLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(quantitylLabel);
// Set up the quantity formatted text field
NumberFormat quantityFormat = NumberFormat.getIntegerInstance();
quantityFormat.setMaximumIntegerDigits(2);
JFormattedTextField quantityTextField = new JFormattedTextField(quantityFormat);
quantityTextField.setColumns(10);
contentPane.add(quantityTextField);
// Set up the date label
JLabel dateLabel = new JLabel("Date", SwingConstants.TRAILING);
dateLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(dateLabel);
// Set up the date formatted text field
MaskFormatter dateMaskFormatter = new MaskFormatter("##/##/####");
JFormattedTextField dateTextField = new JFormattedTextField(dateMaskFormatter);
dateTextField.setColumns(10);
contentPane.add(dateTextField);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
setTitle("FormattedTextFields");
setSize(300, 150);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
try{
new FormattedTextFields();
}
catch(ParseException e){
e.printStackTrace();
}
}
});
}
}
import java.text.NumberFormat;
import java.text.ParseException;
import javax.swing.BorderFactory;
import javax.swing.JFormattedTextField;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
import javax.swing.text.MaskFormatter;
public final class FormattedTextFields extends JFrame {
public FormattedTextFields() throws ParseException {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() throws ParseException {
// Set up the content pane
JPanel contentPane = new JPanel(new GridLayout(0, 2));
contentPane.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
// Set up the price label
JLabel priceLabel = new JLabel("Price", SwingConstants.TRAILING);
priceLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(priceLabel);
// Set up the price formatted text field
NumberFormat priceFormat = NumberFormat.getNumberInstance();
priceFormat.setMaximumFractionDigits(2);
JFormattedTextField priceTextField = new JFormattedTextField(priceFormat);
priceTextField.setColumns(10);
contentPane.add(priceTextField);
// Set up the product reference label
JLabel productReferenceLabel = new JLabel("Product reference", SwingConstants.TRAILING);
productReferenceLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(productReferenceLabel);
// Set up the product reference formatted text field
MaskFormatter productReferenceFormat = new MaskFormatter("###-###-###");
JFormattedTextField productReferenceTextField = new JFormattedTextField(productReferenceFormat);
productReferenceTextField.setColumns(10);
contentPane.add(productReferenceTextField);
// Set up the quantity label
JLabel quantitylLabel = new JLabel("Quantity", SwingConstants.TRAILING);
quantitylLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(quantitylLabel);
// Set up the quantity formatted text field
NumberFormat quantityFormat = NumberFormat.getIntegerInstance();
quantityFormat.setMaximumIntegerDigits(2);
JFormattedTextField quantityTextField = new JFormattedTextField(quantityFormat);
quantityTextField.setColumns(10);
contentPane.add(quantityTextField);
// Set up the date label
JLabel dateLabel = new JLabel("Date", SwingConstants.TRAILING);
dateLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 10));
contentPane.add(dateLabel);
// Set up the date formatted text field
MaskFormatter dateMaskFormatter = new MaskFormatter("##/##/####");
JFormattedTextField dateTextField = new JFormattedTextField(dateMaskFormatter);
dateTextField.setColumns(10);
contentPane.add(dateTextField);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
setTitle("FormattedTextFields");
setSize(300, 150);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
try{
new FormattedTextFields();
}
catch(ParseException e){
e.printStackTrace();
}
}
});
}
}
You are here :
JavaPerspective.com >
Intermediate Tutorials >
5. Graphical User Interfaces >
5.11. Formatted Text Fields
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.12. Password Fields
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.12. Password Fields