5.27. Tabbed panes
Last updated: 1 January 2013.
This tutorial will show you how to use tabbed panes in Java.
A tabbed pane is an instance of the class JTabbedPane. It is a component that allows you to create tabs in a GUI. Each tab is associated with a component that is displayed when the user selects the tab. For example, the following picture shows a frame containing 3 tabs, each of which is associated with a panel:
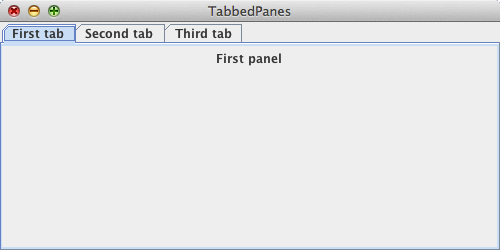
Here is the code that builds the GUI shown above:
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTabbedPane;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
public final class TabbedPanes extends JFrame {
public TabbedPanes() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() {
// Create the tabbed pane
JTabbedPane tabbedPane = new JTabbedPane();
// Set up the first panel
JPanel firstPanel = new JPanel();
firstPanel.add(new JLabel("First panel"));
// Set up the second panel
JPanel secondPanel = new JPanel();
secondPanel.add(new JLabel("Second panel"));
// Set up the third panel
JPanel thirdPanel = new JPanel();
thirdPanel.add(new JLabel("Third panel"));
// Add the panels to the tabbed pane
tabbedPane.addTab("First tab", firstPanel);
tabbedPane.addTab("Second tab", secondPanel);
tabbedPane.addTab("Third tab", thirdPanel);
// Add the tabbed pane to the JFrame
add(tabbedPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("TabbedPanes");
setSize(500, 250);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TabbedPanes();
}
});
}
}
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTabbedPane;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
public final class TabbedPanes extends JFrame {
public TabbedPanes() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() {
// Create the tabbed pane
JTabbedPane tabbedPane = new JTabbedPane();
// Set up the first panel
JPanel firstPanel = new JPanel();
firstPanel.add(new JLabel("First panel"));
// Set up the second panel
JPanel secondPanel = new JPanel();
secondPanel.add(new JLabel("Second panel"));
// Set up the third panel
JPanel thirdPanel = new JPanel();
thirdPanel.add(new JLabel("Third panel"));
// Add the panels to the tabbed pane
tabbedPane.addTab("First tab", firstPanel);
tabbedPane.addTab("Second tab", secondPanel);
tabbedPane.addTab("Third tab", thirdPanel);
// Add the tabbed pane to the JFrame
add(tabbedPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("TabbedPanes");
setSize(500, 250);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TabbedPanes();
}
});
}
}
As you can see, setting up a tabbed pane is quite simple. I just created a JTabbedPane instance and the panels before calling the method addTab(String title, Component component) to set the title and component of each tab. You can remove one or more tabs by calling the method remove(Component component), remove(int index) or removeAll.
By default, the tabs are placed at the top of their container. You can specify a different location by using the constructor JTabbedPane(int tabPlacement). The possible values for the argument tabPlacement are JTabbedPane.TOP, JTabbedPane.LEFT, JTabbedPane.BOTTOM, and JTabbedPane.RIGHT. Alternatively, you can call the method setTabPlacement(int tabPlacement).
If you create numerous tabs, their container may not be wide enough to place them in a single alignment. In that case, you can specify the tab layout policy either by using the constructor JTabbedPane(int tabPlacement, int tabLayoutPolicy) or by calling the method setTabLayoutPolicy(int tabLayoutPolicy). The possible values for the argument tabLayoutPolicy are JTabbedPane.WRAP_TAB_LAYOUT (the default) and JTabbedPane.SCROLL_TAB_LAYOUT.
Note that the class JTabbedPane provides the methods addTab(String title, Icon icon, Component component) and addTab(String title, Icon icon, Component component, String tip) to bind an icon and tool tip text to a tab.
Customization
You can customize the area that displays the title of a tab by calling the method setTabComponentAt(int index, Component component). The argument component is a component that renders the title of the tab. The picture shown below is a GUI that allows you to create tabs dynamically by clicking the button New. Each newly created tab contains a text area in which you can open a text file by clicking the button Open. A text file is opened in the currently selected tab or in a new tab if no tab is selected. The area that displays each tab's title is rendered by a custom component containing the title of the tab and a label (x) that closes the tab when it is clicked.
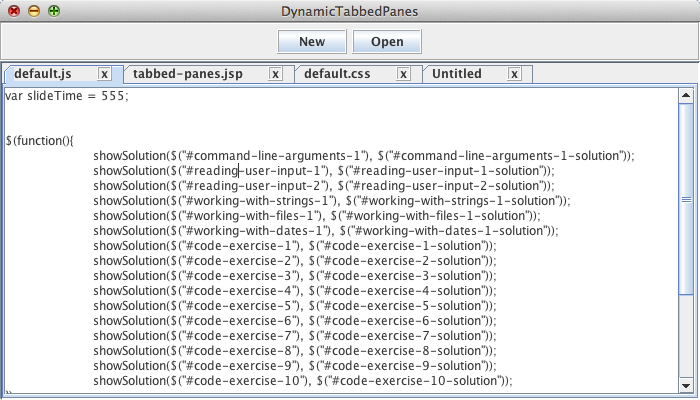
The custom component that renders the title of the tab is a panel whose layout manager is BorderLayout. A label displaying the title is placed at the center and another label displaying the letter x is placed on the right hand side. Here is the code:
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.BorderFactory;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTabbedPane;
import javax.swing.SwingConstants;
import javax.swing.border.BevelBorder;
import javax.swing.border.EtchedBorder;
public final class CustomTabComponent extends JPanel {
private JTabbedPane tabbedPane;
private String title;
public CustomTabComponent(JTabbedPane tabbedPane, String title) {
this.tabbedPane = tabbedPane;
this.title = title;
buildCustomTab();
}
private void buildCustomTab() {
// Set the layout to BorderLayout
setLayout(new BorderLayout());
// Set up the label that displays the title
JLabel titleLabel = new JLabel(title);
titleLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 25));
add(titleLabel, BorderLayout.CENTER);
// Set up the label that closes the tab
final JLabel closeLabel = new JLabel("x", SwingConstants.CENTER);
closeLabel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
closeLabel.setPreferredSize(new Dimension(15, 15));
closeLabel.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e){
tabbedPane.remove(tabbedPane.indexOfTabComponent(CustomTabComponent.this));
}
public void mouseEntered(MouseEvent e){
closeLabel.setForeground(Color.RED);
closeLabel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
}
public void mouseExited(MouseEvent e){
closeLabel.setForeground(Color.BLACK);
closeLabel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
}
});
add(closeLabel, BorderLayout.LINE_END);
}
}
import java.awt.Color;
import java.awt.Dimension;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.BorderFactory;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTabbedPane;
import javax.swing.SwingConstants;
import javax.swing.border.BevelBorder;
import javax.swing.border.EtchedBorder;
public final class CustomTabComponent extends JPanel {
private JTabbedPane tabbedPane;
private String title;
public CustomTabComponent(JTabbedPane tabbedPane, String title) {
this.tabbedPane = tabbedPane;
this.title = title;
buildCustomTab();
}
private void buildCustomTab() {
// Set the layout to BorderLayout
setLayout(new BorderLayout());
// Set up the label that displays the title
JLabel titleLabel = new JLabel(title);
titleLabel.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 25));
add(titleLabel, BorderLayout.CENTER);
// Set up the label that closes the tab
final JLabel closeLabel = new JLabel("x", SwingConstants.CENTER);
closeLabel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
closeLabel.setPreferredSize(new Dimension(15, 15));
closeLabel.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e){
tabbedPane.remove(tabbedPane.indexOfTabComponent(CustomTabComponent.this));
}
public void mouseEntered(MouseEvent e){
closeLabel.setForeground(Color.RED);
closeLabel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
}
public void mouseExited(MouseEvent e){
closeLabel.setForeground(Color.BLACK);
closeLabel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
}
});
add(closeLabel, BorderLayout.LINE_END);
}
}
The code that builds the GUI follows:
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTabbedPane;
import javax.swing.JTextArea;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.border.BevelBorder;
import javax.swing.border.EtchedBorder;
public final class DynamicTabbedPanes extends JFrame implements ActionListener {
private JButton newButton;
private JButton openButton;
private JTabbedPane tabbedPane;
private JFileChooser fileChooser;
public DynamicTabbedPanes() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if(e.getSource().equals(newButton)) {
tabbedPane.addTab("", new JScrollPane(new JTextArea()));
tabbedPane.setTabComponentAt(tabbedPane.getTabCount() - 1, new CustomTabComponent(tabbedPane, "Untitled"));
}
else if(e.getSource().equals(openButton)){
// Show the file chooser
int choice = fileChooser.showOpenDialog(this);
if(choice == JFileChooser.APPROVE_OPTION){
// Get the selected file
File selectedFile = fileChooser.getSelectedFile();
try{
JTextArea textArea;
if(tabbedPane.getTabCount() > 0){ // Set up the currently selected tab
int index = tabbedPane.getSelectedIndex();
JScrollPane scrollPane = (JScrollPane) tabbedPane.getComponentAt(index);
textArea = (JTextArea) scrollPane.getViewport().getView();
tabbedPane.setTabComponentAt(index, new CustomTabComponent(tabbedPane, selectedFile.getName()));
}
else{ // Set up a new tab
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
tabbedPane.addTab("", scrollPane);
tabbedPane.setTabComponentAt(0, new CustomTabComponent(tabbedPane, selectedFile.getName()));
}
// Open the file
BufferedReader reader = new BufferedReader(new FileReader(selectedFile));
// Display the file's content in the text area
textArea.setText("");
for(String line = reader.readLine(); line != null; line = reader.readLine())
textArea.append(line + "\n");
// Close the reader
reader.close();
}
catch(Exception ex){
ex.printStackTrace();
}
}
}
}
private JButton addButton(String name, Container container, int width, int height){
// Create the button
JButton button = new JButton(name);
// Set the preferred size to the specified value and set up the border
button.setPreferredSize(new Dimension(width, height));
button.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
// Add the button to the container
container.add(button);
// Return the button
return button;
}
private void addComponents() {
// Create the tabbed pane and the file chooser
tabbedPane = new JTabbedPane();
fileChooser = new JFileChooser();
// Set up the content pane
JPanel contentPane = new JPanel();
contentPane.setLayout(new BorderLayout());
// Set up the buttons panel ----------------------------------------------------------------------------
JPanel buttonsPanel = new JPanel(); // Leave the layout to the default FlowLayout
buttonsPanel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.LOWERED));
int buttonWidth = 70;
int buttonHeight = 25;
newButton = addButton("New", buttonsPanel, buttonWidth, buttonHeight);
openButton = addButton("Open", buttonsPanel, buttonWidth, buttonHeight);
newButton.addActionListener(this);
openButton.addActionListener(this);
contentPane.add(buttonsPanel, BorderLayout.PAGE_START);
// -------------------------------------------------------------------------------------------------------
// Set the border of the tabbed pane and add it to the content pane
tabbedPane.setBorder(BorderFactory.createBevelBorder(BevelBorder.LOWERED));
contentPane.add(tabbedPane, BorderLayout.CENTER);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("DynamicTabbedPanes");
setSize(700, 400);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new DynamicTabbedPanes();
}
});
}
}
import java.awt.Container;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTabbedPane;
import javax.swing.JTextArea;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.border.BevelBorder;
import javax.swing.border.EtchedBorder;
public final class DynamicTabbedPanes extends JFrame implements ActionListener {
private JButton newButton;
private JButton openButton;
private JTabbedPane tabbedPane;
private JFileChooser fileChooser;
public DynamicTabbedPanes() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if(e.getSource().equals(newButton)) {
tabbedPane.addTab("", new JScrollPane(new JTextArea()));
tabbedPane.setTabComponentAt(tabbedPane.getTabCount() - 1, new CustomTabComponent(tabbedPane, "Untitled"));
}
else if(e.getSource().equals(openButton)){
// Show the file chooser
int choice = fileChooser.showOpenDialog(this);
if(choice == JFileChooser.APPROVE_OPTION){
// Get the selected file
File selectedFile = fileChooser.getSelectedFile();
try{
JTextArea textArea;
if(tabbedPane.getTabCount() > 0){ // Set up the currently selected tab
int index = tabbedPane.getSelectedIndex();
JScrollPane scrollPane = (JScrollPane) tabbedPane.getComponentAt(index);
textArea = (JTextArea) scrollPane.getViewport().getView();
tabbedPane.setTabComponentAt(index, new CustomTabComponent(tabbedPane, selectedFile.getName()));
}
else{ // Set up a new tab
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
tabbedPane.addTab("", scrollPane);
tabbedPane.setTabComponentAt(0, new CustomTabComponent(tabbedPane, selectedFile.getName()));
}
// Open the file
BufferedReader reader = new BufferedReader(new FileReader(selectedFile));
// Display the file's content in the text area
textArea.setText("");
for(String line = reader.readLine(); line != null; line = reader.readLine())
textArea.append(line + "\n");
// Close the reader
reader.close();
}
catch(Exception ex){
ex.printStackTrace();
}
}
}
}
private JButton addButton(String name, Container container, int width, int height){
// Create the button
JButton button = new JButton(name);
// Set the preferred size to the specified value and set up the border
button.setPreferredSize(new Dimension(width, height));
button.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
// Add the button to the container
container.add(button);
// Return the button
return button;
}
private void addComponents() {
// Create the tabbed pane and the file chooser
tabbedPane = new JTabbedPane();
fileChooser = new JFileChooser();
// Set up the content pane
JPanel contentPane = new JPanel();
contentPane.setLayout(new BorderLayout());
// Set up the buttons panel ----------------------------------------------------------------------------
JPanel buttonsPanel = new JPanel(); // Leave the layout to the default FlowLayout
buttonsPanel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.LOWERED));
int buttonWidth = 70;
int buttonHeight = 25;
newButton = addButton("New", buttonsPanel, buttonWidth, buttonHeight);
openButton = addButton("Open", buttonsPanel, buttonWidth, buttonHeight);
newButton.addActionListener(this);
openButton.addActionListener(this);
contentPane.add(buttonsPanel, BorderLayout.PAGE_START);
// -------------------------------------------------------------------------------------------------------
// Set the border of the tabbed pane and add it to the content pane
tabbedPane.setBorder(BorderFactory.createBevelBorder(BevelBorder.LOWERED));
contentPane.add(tabbedPane, BorderLayout.CENTER);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("DynamicTabbedPanes");
setSize(700, 400);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new DynamicTabbedPanes();
}
});
}
}
You are here :
JavaPerspective.com >
Intermediate Tutorials >
5. Graphical User Interfaces >
5.27. Tabbed panes
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.28. Color choosers
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.28. Color choosers