5.23. Separators
Last updated: 1 January 2013.
This tutorial will show you how to use separators in Java.
As its name implies, a separator is a horizontal or vertical line or an empty space that separates components. You may have already seen separators in the tutorials Menus and Tool bars. The easiest way to add a separator to a menu or tool bar is to call the method addSeparator provided by the classes JMenu, JPopupMenu and JToolBar. As an example, the next picture shows a menu with several horizontal separators:

The separators in the above picture were added to the menu by calling the method addSeparator provided by the class JMenu. Similarly, the picture shown below is a frame containing a tool bar with several buttons and a separator. In this case, the separator is a vertical empty space (between the blue and yellow button) that was added by a call to the method addSeparator provided by the class JToolBar.
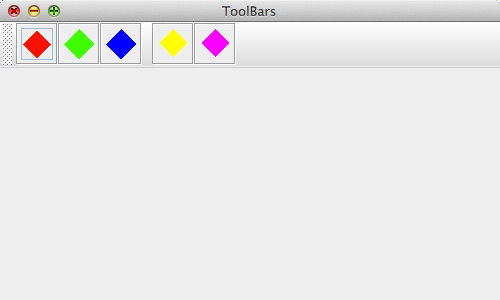
The code that builds the frames shown above can be found in the tutorials Menus and Tool bars.
The class JSeparator
The class JSeparator allows you to use line separators with all kinds of containers, including menus and tool bars. However, the method addSeparator is the preferred way of adding separators to menus and tool bars as explained earlier. The following picture shows a frame with 3 buttons, a vertical line separator and a text field:

Here is the code:
import java.awt.Dimension;
import javax.swing.BorderFactory;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JSeparator;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
public final class Separators extends JFrame {
public Separators() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() {
// Set up the content pane
JPanel contentPane = new JPanel();
contentPane.setLayout(new BoxLayout(contentPane, BoxLayout.LINE_AXIS));
contentPane.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
// Set up button 1
JButton button1 = new JButton("Button 1");
contentPane.add(button1);
// Set up button 2
JButton button2 = new JButton("Button 2");
contentPane.add(button2);
// Set up button 3
JButton button3 = new JButton("Button 3");
contentPane.add(button3);
// Add space between button 3 and the separator
contentPane.add(Box.createRigidArea(new Dimension(10,0)));
// Set up the separator
JSeparator separator = new JSeparator(SwingConstants.VERTICAL);
contentPane.add(separator);
// Add space between the separator and the text field
contentPane.add(Box.createRigidArea(new Dimension(10,0)));
// Set up the text field
JTextField textField = new JTextField(7);
contentPane.add(textField);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
setTitle("Separators");
setSize(500, 80);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new Separators();
}
});
}
}
import javax.swing.BorderFactory;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JSeparator;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
public final class Separators extends JFrame {
public Separators() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() {
// Set up the content pane
JPanel contentPane = new JPanel();
contentPane.setLayout(new BoxLayout(contentPane, BoxLayout.LINE_AXIS));
contentPane.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
// Set up button 1
JButton button1 = new JButton("Button 1");
contentPane.add(button1);
// Set up button 2
JButton button2 = new JButton("Button 2");
contentPane.add(button2);
// Set up button 3
JButton button3 = new JButton("Button 3");
contentPane.add(button3);
// Add space between button 3 and the separator
contentPane.add(Box.createRigidArea(new Dimension(10,0)));
// Set up the separator
JSeparator separator = new JSeparator(SwingConstants.VERTICAL);
contentPane.add(separator);
// Add space between the separator and the text field
contentPane.add(Box.createRigidArea(new Dimension(10,0)));
// Set up the text field
JTextField textField = new JTextField(7);
contentPane.add(textField);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
setTitle("Separators");
setSize(500, 80);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new Separators();
}
});
}
}
As you can see, I did not set the size of the separator because I used BoxLayout as a layout manager and thereby left the sizing of the separator to BoxLayout. If you do not specify a layout manager, you need to set the size of the separator explicitly because the preferred width of a horizontal separator is 0 by default and the preferred height of a vertical separator is 0 by default. To set the size of a separator, call the methods setPreferredSize(Dimension preferredSize), setMinimumSize(Dimension minimumSize) and setMaximumSize(Dimension maximumSize).
You are here :
JavaPerspective.com >
Intermediate Tutorials >
5. Graphical User Interfaces >
5.23. Separators
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.24. Internal frames
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.24. Internal frames