5.29. Sliders
Last updated: 1 January 2013.
This tutorial will show you how to use sliders in Java.
A slider is an instance of the class JSlider. It is a component that allows the user to select a number from a given interval by sliding a knob. A slider can be either horizontal or vertical. As an example, the following picture shows a frame containing a text area whose font size is set by a horizontal slider placed at the bottom of the frame:

The code that builds this GUI is shown below:
import java.awt.BorderLayout;
import java.awt.Font;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSlider;
import javax.swing.JTextArea;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
public final class Sliders extends JFrame implements ChangeListener {
private JTextArea textArea;
private JSlider fontSizeSlider;
private Font font = new Font("Times New Roman", Font.PLAIN, 25);
private final int MIN_FONT_SIZE = 15;
private final int MAX_FONT_SIZE = 75;
private final int INITIAL_FONT_SIZE = 25;
public Sliders() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() {
// Create the content pane
JPanel contentPane = new JPanel(new BorderLayout());
// Set up the text area
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
contentPane.add(scrollPane);
// Create the slider
fontSizeSlider = new JSlider(SwingConstants.HORIZONTAL, MIN_FONT_SIZE, MAX_FONT_SIZE, INITIAL_FONT_SIZE);
// Decorate the slider with ticks and set labels at major tick marks
fontSizeSlider.setMinorTickSpacing(1);
fontSizeSlider.setMajorTickSpacing(5);
fontSizeSlider.setPaintTicks(true);
fontSizeSlider.setPaintLabels(true);
// Add a change listener to the slider
fontSizeSlider.addChangeListener(this);
// Add the slider to the content pane
contentPane.add(fontSizeSlider, BorderLayout.PAGE_END);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("Sliders");
setSize(700, 400);
setLocationRelativeTo(null);
}
public void stateChanged(ChangeEvent e){
JSlider source = (JSlider) e.getSource();
if(! source.getValueIsAdjusting()){
textArea.setFont(font.deriveFont((float) source.getValue()));
}
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new Sliders();
}
});
}
}
import java.awt.Font;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSlider;
import javax.swing.JTextArea;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
public final class Sliders extends JFrame implements ChangeListener {
private JTextArea textArea;
private JSlider fontSizeSlider;
private Font font = new Font("Times New Roman", Font.PLAIN, 25);
private final int MIN_FONT_SIZE = 15;
private final int MAX_FONT_SIZE = 75;
private final int INITIAL_FONT_SIZE = 25;
public Sliders() {
init();
addComponents();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void addComponents() {
// Create the content pane
JPanel contentPane = new JPanel(new BorderLayout());
// Set up the text area
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
contentPane.add(scrollPane);
// Create the slider
fontSizeSlider = new JSlider(SwingConstants.HORIZONTAL, MIN_FONT_SIZE, MAX_FONT_SIZE, INITIAL_FONT_SIZE);
// Decorate the slider with ticks and set labels at major tick marks
fontSizeSlider.setMinorTickSpacing(1);
fontSizeSlider.setMajorTickSpacing(5);
fontSizeSlider.setPaintTicks(true);
fontSizeSlider.setPaintLabels(true);
// Add a change listener to the slider
fontSizeSlider.addChangeListener(this);
// Add the slider to the content pane
contentPane.add(fontSizeSlider, BorderLayout.PAGE_END);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("Sliders");
setSize(700, 400);
setLocationRelativeTo(null);
}
public void stateChanged(ChangeEvent e){
JSlider source = (JSlider) e.getSource();
if(! source.getValueIsAdjusting()){
textArea.setFont(font.deriveFont((float) source.getValue()));
}
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new Sliders();
}
});
}
}
A slider has minor ticks and major ticks. The method setMinorTickSpacing(int n) sets the space between minor tick marks. Likewise, the method setMajorTickSpacing(int n) sets the space between major tick marks. For the ticks to be visible, you must make the call setPaintTicks(true). The call setPaintLabels(true) displays labels at each major tick mark.
As you can see, in order to listen to changes in the slider, I added a change listener to it. The method getValueIsAdjusting is to ensure that the user has finished sliding the knob.
You can customize the labels of a slider by creating a Hashtable containing key-value pairs. Each key is an integer representing a tick within the slider's interval and each value is a label that is placed at the corresponding tick. The method setLabelTable(Dictionary labels) sets the label table to use. Here is an example:
// Decorate the slider with ticks and set custom labels at tick marks
fontSizeSlider.setMajorTickSpacing(20);
fontSizeSlider.setPaintTicks(true);
Hashtable<Integer, JLabel> labelsTable = new Hashtable<Integer, JLabel>();
labelsTable.put(15, new JLabel("Small"));
labelsTable.put(35, new JLabel("Average"));
labelsTable.put(55, new JLabel("Big"));
labelsTable.put(75, new JLabel("Bigger"));
fontSizeSlider.setLabelTable(labelsTable);
fontSizeSlider.setPaintLabels(true);
fontSizeSlider.setMajorTickSpacing(20);
fontSizeSlider.setPaintTicks(true);
Hashtable<Integer, JLabel> labelsTable = new Hashtable<Integer, JLabel>();
labelsTable.put(15, new JLabel("Small"));
labelsTable.put(35, new JLabel("Average"));
labelsTable.put(55, new JLabel("Big"));
labelsTable.put(75, new JLabel("Bigger"));
fontSizeSlider.setLabelTable(labelsTable);
fontSizeSlider.setPaintLabels(true);
Here is the modified GUI:
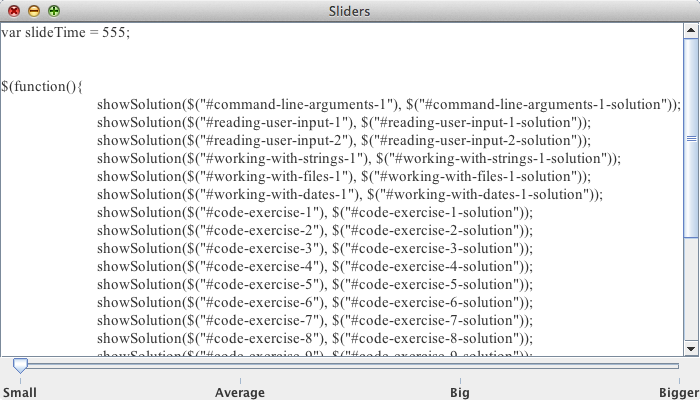
You are here :
JavaPerspective.com >
Intermediate Tutorials >
5. Graphical User Interfaces >
5.29. Sliders
Next tutorial : JavaPerspective.com > Advanced Tutorials
Next tutorial : JavaPerspective.com > Advanced Tutorials