5.26. File choosers
Last updated: 5 January 2013.
This tutorial will show you how to use file choosers in Java.
A file chooser is an instance of the class JFileChooser. It is a modal dialog that lets the user select a file or directory from the file system. To display a file chooser, all you need to do is create an instance of the class JFileChooser and call upon it one of the following methods:
- showOpenDialog(Component parent): Displays a file chooser with the title Open, containing two buttons named Open and Cancel.
- showSaveDialog(Component parent): Displays a file chooser with the title Save, containing two buttons named Save and Cancel.
- showDialog(Component parent, String approveButtonText): Displays a file chooser with the specified title approveButtonText, containing two buttons named approveButtonText and Cancel.
5.26.1. The method showOpenDialog
The following sample displays a file chooser by calling the method showOpenDialog(Component parent):
JFileChooser fileChooser = new JFileChooser();
int choice = fileChooser.showOpenDialog(parent);
int choice = fileChooser.showOpenDialog(parent);
In the above sample, parent is an instance of the class JFrame. The no-argument constructor JFileChooser() creates a file chooser that shows the user's home directory the first time it is displayed. You can specify another directory by using other constructors or by calling the method setCurrentDirectory(File dir). Here is the displayed file chooser:

As you can see, the approve button is named Open. Nonetheless, clicking that button does not actually open a file but only selects it. Your code must handle the selected file appropriately. For example, if you want to open a text file, you have to read its content with a class like FileReader or BufferedReader and display that content in a text area as shown in the last code sample of this tutorial. You can get the selected file by calling the method getSelectedFile as shown below:
JFileChooser fileChooser = new JFileChooser();
int choice = fileChooser.showOpenDialog(parent);
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
int choice = fileChooser.showOpenDialog(parent);
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
5.26.2. The method showSaveDialog
The method showSaveDialog(Component parent) is used to create file choosers that look pretty much the same as the dialog shown in the previous section. The only difference is the title and the name of the approve button. For example, here is a sample that uses the method showSaveDialog(Component parent):
JFileChooser fileChooser = new JFileChooser();
int choice = fileChooser.showSaveDialog(parent);
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
int choice = fileChooser.showSaveDialog(parent);
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
Here is the file chooser:
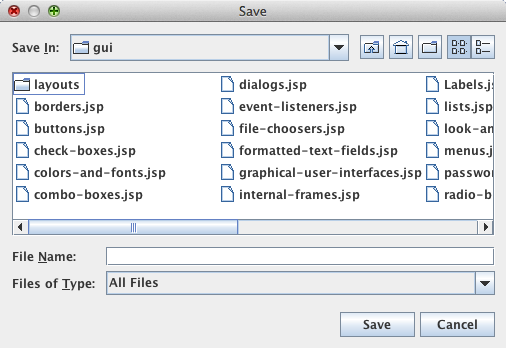
Of course, the button save does not save a file but only selects it. If you want to save the selected file, you have to use classes like FileWriter or BufferedWriter. The last sample in this tutorial will show you how to use a file chooser to save a text file in a directory.
5.26.3. The method showDialog
The method showDialog(Component parent, String approveButtonText) comes in handy when you want to perform a task other than opening or saving a file. You can set a custom text on the approve button an also a custom title to indicate the kind of operation that is performed on the selected file. Here is a sample that creates a file chooser whose purpose would be to compress the selected file:
JFileChooser fileChooser = new JFileChooser();
int choice = fileChooser.showDialog(parent, "Compress");
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
int choice = fileChooser.showDialog(parent, "Compress");
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
The created file chooser is shown below:
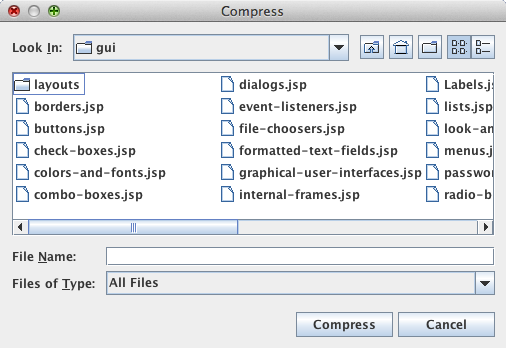
5.26.4. Other interesting methods
The class JFileChooser provides other useful methods like setMultiSelectionEnabled(boolean b) that lets the user select multiple files at a time. You might also want to use the method setFileSelectionMode(int mode) which allows you to select just files, just directories or both. The possible values for the argument mode are JFileChooser.FILES_ONLY, JFileChooser.DIRECTORIES_ONLY and JFileChooser.FILES_AND_DIRECTORIES.
Last but not least, the method setFileFilter(FileFilter filter) applies a filter to a file chooser. For example, you can show in a file chooser only the files that have the extensions .java, .js, .jsp and .css like this:
FileFilter filter = new FileNameExtensionFilter("Java, Javascript, JSP and CSS files", "java", "js", "jsp", "css");
JFileChooser fileChooser = new JFileChooser();
fileChooser.setFileFilter(filter);
int choice = fileChooser.showOpenDialog(parent);
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
JFileChooser fileChooser = new JFileChooser();
fileChooser.setFileFilter(filter);
int choice = fileChooser.showOpenDialog(parent);
if(choice == JFileChooser.APPROVE_OPTION){
File selectedFile = fileChooser.getSelectedFile();
// Process the selected file appropriately
// ...
}
else{
// The user has cancelled the operation
}
5.26.5. How to open and save a file with a file chooser
This section gives you tips in opening a file in a text area and saving the content of a text area into a text file. The next picture is a snapshot of a frame containing two buttons at the top (Open and Save), a text area at the center and a label at the bottom. When the user clicks the Open button, a file chooser pops up to let the user select a file whose content is displayed in the text area. The Save button allows the user to save the content of the text area into a file. Be careful not to overwrite existing files if you run this basic example (the program will not warn you before overwriting a file). Finally, the label at the bottom of the frame displays the absolute path of the current file.

The code follows:
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.border.EtchedBorder;
public final class FileChoosers extends JFrame implements ActionListener {
private JButton openButton;
private JButton saveButton;
private JTextArea textArea;
private JLabel absolutePathLabel;
private JFileChooser fileChooser;
public FileChoosers() {
init();
addComponents();
fileChooser = new JFileChooser();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if(e.getSource().equals(openButton)){
// Show the open file chooser
int choice = fileChooser.showOpenDialog(this);
if(choice == JFileChooser.APPROVE_OPTION){
// Get the selected file
File selectedFile = fileChooser.getSelectedFile();
// Open the file
try{
textArea.setText("");
BufferedReader reader = new BufferedReader(new FileReader(selectedFile));
for(String line = reader.readLine(); line != null; line = reader.readLine())
textArea.append(line + "\n");
reader.close();
}
catch(Exception ex){
ex.printStackTrace();
}
// Set the bottom label's text to the absolute path of the selected file
absolutePathLabel.setText(selectedFile.getAbsolutePath());
}
}
else if(e.getSource().equals(saveButton)){
// Show the save file chooser
int choice = fileChooser.showSaveDialog(this);
if(choice == JFileChooser.APPROVE_OPTION){
// Get the selected file
File selectedFile = fileChooser.getSelectedFile();
// Save the file
try{
BufferedWriter writer = new BufferedWriter(new FileWriter(selectedFile));
writer.write(textArea.getText());
writer.close();
}
catch(Exception ex){
ex.printStackTrace();
}
// Set the bottom label's text to the absolute path of the selected file
absolutePathLabel.setText(selectedFile.getAbsolutePath());
}
}
}
private JButton addButton(String name, Container container, int width, int height){
// Create the button
JButton button = new JButton(name);
// Set the preferred size to the specified value
button.setPreferredSize(new Dimension(width, height));
button.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
// Add the button to the container
container.add(button);
// Return the button
return button;
}
private void addComponents() {
// Set up the content pane
JPanel contentPane = new JPanel();
contentPane.setLayout(new BorderLayout());
// Set up the buttons panel ---------------------------------------------------------------------
JPanel buttonsPanel = new JPanel(); // Leave the layout to the default FlowLayout
int buttonWidth = 70;
int buttonHeight = 25;
openButton = addButton("Open", buttonsPanel, buttonWidth, buttonHeight);
saveButton = addButton("Save", buttonsPanel, buttonWidth, buttonHeight);
openButton.addActionListener(this);
saveButton.addActionListener(this);
contentPane.add(buttonsPanel, BorderLayout.PAGE_START);
// -------------------------------------------------------------------------------------------------
// Set up the text area
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
contentPane.add(scrollPane, BorderLayout.CENTER);
// Set up the bottom label
absolutePathLabel = new JLabel();
contentPane.add(absolutePathLabel, BorderLayout.PAGE_END);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("FileChoosers");
setSize(700, 400);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FileChoosers();
}
});
}
}
import java.awt.Container;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.border.EtchedBorder;
public final class FileChoosers extends JFrame implements ActionListener {
private JButton openButton;
private JButton saveButton;
private JTextArea textArea;
private JLabel absolutePathLabel;
private JFileChooser fileChooser;
public FileChoosers() {
init();
addComponents();
fileChooser = new JFileChooser();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if(e.getSource().equals(openButton)){
// Show the open file chooser
int choice = fileChooser.showOpenDialog(this);
if(choice == JFileChooser.APPROVE_OPTION){
// Get the selected file
File selectedFile = fileChooser.getSelectedFile();
// Open the file
try{
textArea.setText("");
BufferedReader reader = new BufferedReader(new FileReader(selectedFile));
for(String line = reader.readLine(); line != null; line = reader.readLine())
textArea.append(line + "\n");
reader.close();
}
catch(Exception ex){
ex.printStackTrace();
}
// Set the bottom label's text to the absolute path of the selected file
absolutePathLabel.setText(selectedFile.getAbsolutePath());
}
}
else if(e.getSource().equals(saveButton)){
// Show the save file chooser
int choice = fileChooser.showSaveDialog(this);
if(choice == JFileChooser.APPROVE_OPTION){
// Get the selected file
File selectedFile = fileChooser.getSelectedFile();
// Save the file
try{
BufferedWriter writer = new BufferedWriter(new FileWriter(selectedFile));
writer.write(textArea.getText());
writer.close();
}
catch(Exception ex){
ex.printStackTrace();
}
// Set the bottom label's text to the absolute path of the selected file
absolutePathLabel.setText(selectedFile.getAbsolutePath());
}
}
}
private JButton addButton(String name, Container container, int width, int height){
// Create the button
JButton button = new JButton(name);
// Set the preferred size to the specified value
button.setPreferredSize(new Dimension(width, height));
button.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.RAISED));
// Add the button to the container
container.add(button);
// Return the button
return button;
}
private void addComponents() {
// Set up the content pane
JPanel contentPane = new JPanel();
contentPane.setLayout(new BorderLayout());
// Set up the buttons panel ---------------------------------------------------------------------
JPanel buttonsPanel = new JPanel(); // Leave the layout to the default FlowLayout
int buttonWidth = 70;
int buttonHeight = 25;
openButton = addButton("Open", buttonsPanel, buttonWidth, buttonHeight);
saveButton = addButton("Save", buttonsPanel, buttonWidth, buttonHeight);
openButton.addActionListener(this);
saveButton.addActionListener(this);
contentPane.add(buttonsPanel, BorderLayout.PAGE_START);
// -------------------------------------------------------------------------------------------------
// Set up the text area
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
contentPane.add(scrollPane, BorderLayout.CENTER);
// Set up the bottom label
absolutePathLabel = new JLabel();
contentPane.add(absolutePathLabel, BorderLayout.PAGE_END);
// Add the content pane to the JFrame
add(contentPane);
}
private void init() {
try{
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());
}
catch(Exception e){
e.printStackTrace();
}
setTitle("FileChoosers");
setSize(700, 400);
setLocationRelativeTo(null);
}
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FileChoosers();
}
});
}
}
You are here :
JavaPerspective.com >
Intermediate Tutorials >
5. Graphical User Interfaces >
5.26. File choosers
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.27. Tabbed panes
Next tutorial : JavaPerspective.com > Intermediate Tutorials > 5. Graphical User Interfaces > 5.27. Tabbed panes