1.2. How does Java work?
Last updated: 23 January 2013.
Java is a platform independent, object-oriented and class-based programming language.
1.1. Java is platform independent
Java is a Write Once Run Anywhere programming language (WORA), which means that a Java program can be compiled on Linux and run on Windows. That platform independence is achieved by compiling the Java source code into a generic format called bytecode. Then a piece of software known as the Java Virtual Machine (JVM) converts the bytecode to native code and executes it. In fact, the JVM is a Java bytecode interpreter that is written in the native code of the host computer.
Let's say you have written a Java program called SaveFile.java that creates a new file and saves it into a folder. The picture below shows how it works:
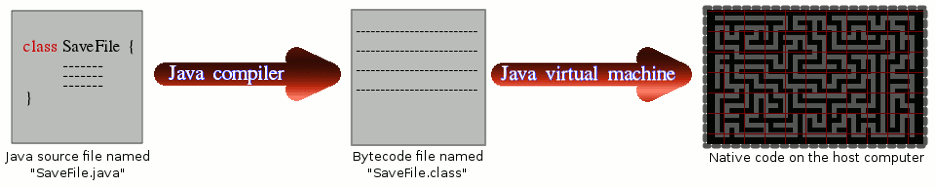
Because file reading and writing are low-level operations performed through services provided by the operating system kernel, at some point, in order to save the file, the JVM has to make an operating system call to the appropriate kernel service. Therefore, Java comes along with standard libraries that allow, among other things, such an operating system call. Those libraries are known as the Java API (Application Programming Interface). The Java API and the JVM altogether are called the Java Runtime Environment (JRE). The JRE is also simply termed the Java platform.
Thus, if you want to run Java programs, a JRE must be installed on your device. If you also want to compile Java source code, you'll need a Java Development Kit (JDK) which features a compiler and other development tools. An important point to note is that the JRE is included in the JDK. Consequently, if you want to compile and run Java programs, all you have to do is install the JDK. The word SDK (Standard Development Kit) is sometimes used to refer to the JDK plus additional software, such as debuggers, application servers and documentation.
1.2. Java is an object-oriented programming language
An object-oriented programming language is a language that tries to represent the real world in a computer's memory by making these simple statements:
- Everything can be represented as an object.
- An object can be created and destroyed.
- At a given time, an object has a status that can be modified.
- Every object has its own behaviour.

As you can see from the above picture, the object has a status which is its location (x, y). At a given time, x and y have values that can be modified. Moreover, the object has its own behaviour since it can display its location onscreen and even move to another location.
Now how to create and destroy objects?
An object is created by allocating memory to it. Conversely, an object is destroyed by freeing its allocated memory, thereby making that memory available again for other purposes. Fortunately, memory management is an automated process in Java. Hence, Java developers do not have to allocate or free the memory of the objects they use.
1.3. Java is a class-based programming language
Every piece of Java code must be inside a class. In the Java language, everything revolves around classes. A class is the code representation of an object, that is, if you want to create an object in memory, the first thing to do is to write its code in a class and save it to a text file with the extension ".java" (there is one file per object).
A class contains fields and functions called methods. The fields are the status of the class and the methods determine the behaviour of the class. A method is a series of statements enclosed in curly braces. As an example, the class representing a point in the cartesian coordinate system will look something like this:
class Point {
private int x;
private int y;
public void show() {
// the statements to display the coordinates go here
}
public void move(int x, int y){
// the statements to change the coordinates go here
}
}
private int x;
private int y;
public void show() {
// the statements to display the coordinates go here
}
public void move(int x, int y){
// the statements to change the coordinates go here
}
}
The class Point has two fields (x and y) and two methods (show and move). For now, understanding the code above is not necessary. There will be more code explanation later.
You are here :
JavaPerspective.com >
Beginner Tutorials >
1. What is Java? >
1.2. How does Java work?
Next tutorial : JavaPerspective.com > Beginner Tutorials > 2. Getting Started
Next tutorial : JavaPerspective.com > Beginner Tutorials > 2. Getting Started